2019 Mar 05
Tactics RPG - Stats and Levels
Shifting gears a little bit from the last post, I'd like to go over characters stats and levels a bit.
Stats
Copied from the enum:
public enum StatTypes {
LVL, // Level
EXP, // Experience
HP, // Hit points
MHP, // Max Hit points
MP, // Magic Points
MMP, // Max Magic Points
SP, // Skill points
MSP, // Max Skill points
INT, // Intelligence
ACC, // Accuracy
ATK, // Attack
DEF, // Defense
MAG, // Magic attack
RES, // Magic resistance
EVD, // Evasion
SPD, // Speed
LCK, // Luck
MOV, // Movement range
JMP, // Jump Height
}
These aren’t final, just some rough ideas I had during development:
Level
Level is, obviously, tied to experience. Currently using the Ease In Quad formula, such that:
- (0, max experience and level percent) are input arguments
- Level percent is Mathf.Clamp01((float)(level - minLevel) / (float)(maxLevel - minLevel));
- Max level is 99
- Min level is 1
Not set in stone, just a basic placeholder until I decide on something else Mainly contained in Rank.cs
Programming TacticsRPG
2019 Mar 04
Fire Emblem CiphAR - Community Feedback
Thank you for all the great feedback! Here is a consolidated list of the bugs found and features requested:
Bugs
1) Text jitter - When scanning some cards, there is a lot of jitter when viewing the text. I'm pretty sure this is because of ARCore re-adjusting the text position relative to the card once per frame, which causes even tiny camera movements to cause the text to shift. I'll probably solve this by implementing a minimum distance moved parameter for the scanned cards before the text is re-adjusted.
2) Cards missing - It looks like my web scraper missed some cards when compiling the image and translation databases. I'll look into these and either fix the issues in the web scraper, or add the missing cards in manually.
3) Text rendering too small - Sometimes, the app mis-approximates the distance of the camera to the card, causing the Y coordinate of the text to be too far away from the camera, making the overlaid text look very small. Still looking into a fix for this.
Features
1) Font changes - A lot of people had readability issues with the default white font color. To alleviate this, I'll implement a menu that can be used to change the font color, font size and font border.
2) Card freezing - Being able to tap a card to freeze its translation in place, so even large camera movements do not shift the translation.
3) Card DB lookup - a separate screen for being able to look up existing translations, without having to scan a card first.
I'll look into fixing most of the bugs this week, then on implementing the new features.
Programming ciphAR
2019 Mar 03
Fire Emblem CiphAR - Android Store Release
The first release of Fire Emblem CiphAR has been rolled out to the Google play store! Here is the store link.
The application was made using Unity3D and ARCore. The image data and card translations were all obtained from Serenes Forest here, and scraped using Python + BeautifulSoup.
Here are some images of the app in use:
An example of a single card being scanned
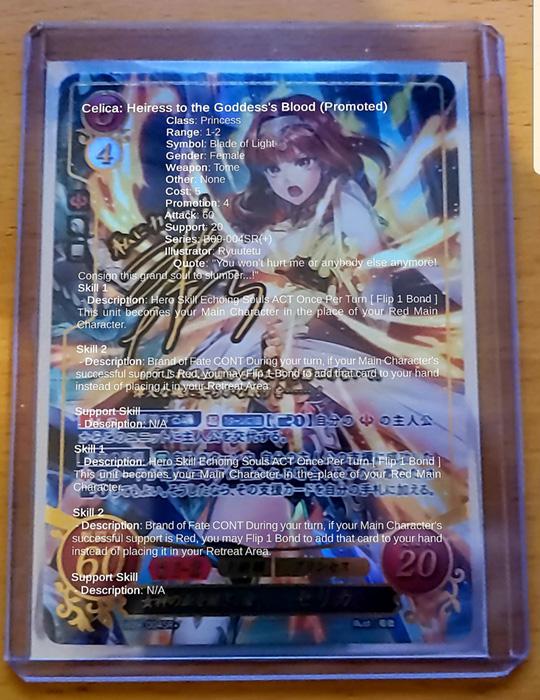
An example with multiple cards being scanned in a full gameplay environment
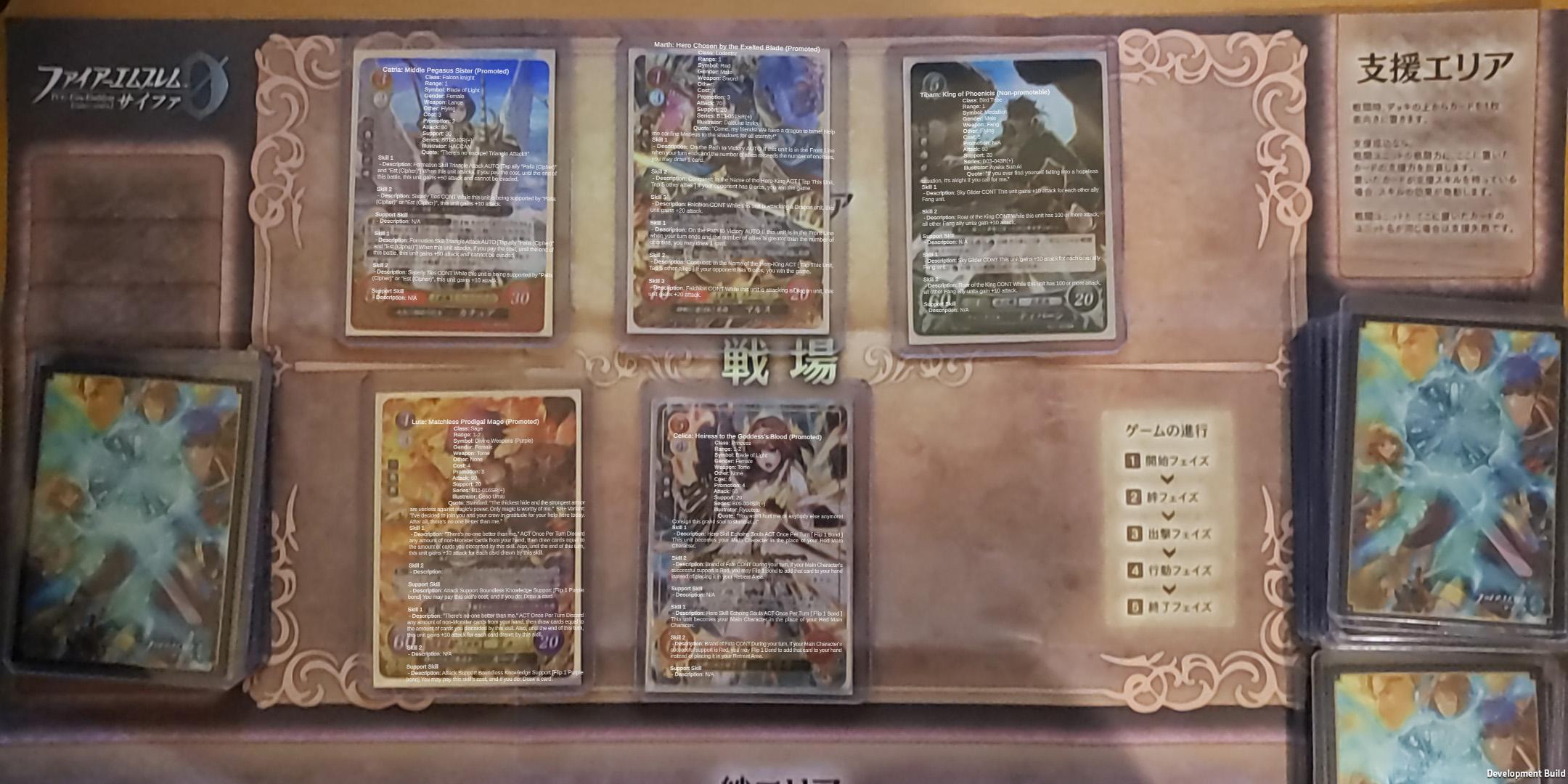
Here's the final logo I decided to go with:
The sword is supposed to be a minimalist Falchion. The 5 circles are meant to be the orbs on the back of most cipher cards (or the orbs in a summoning circle for Fire Emblem Heroes, if you play that). I'm sure none of this actually comes across effectively.
Current issues:
-
There's some jitter in the overlaid text, probably due to ARCore readjusting the position of the text per frame, even on small camera movements.
-
If the card isn't scanned accurately, sometimes it will "fly away" (hard to explain, but you'll know when it happens).
Programming ciphAR
2019 Mar 02
Tactics RPG - Game board representation
One of the most important things to take into consideration when developing a Tactics RPG is the board representation, since almost every other component in your game will have to interact with it in some way.
In my game, a board is composed of individual Tiles. Each Tile has its own attributes, such as its Tile Type, material and texture. In addition, tiles also maintain a reference to the content placed on top of them, such as a player unit, enemy unit or item.
We define a Point on the board as an x,y,z coordinate. It should be noted that here, the y coordinate of the point actually corresponds to the z coordinate in Unity.
Finally, we represent a Board as a Dictionary that maps a Point to a Stack of Tiles. This representation allows me to have multiple Tiles stacked on top of each other on the same Point, while also giving me access to the top Tile on a Point in constant time. This is important for various functions in the game, such as path finding and movement. Having a stack of tiles with potentially different types allows for various interesting mechanics involving terrain transformation, such as terrain destruction and building.
One further optimization made to the above board representation is, if two of the same types of tile are stacked on top of each other, to increase the height of the base tile instead of stacking the two tiles on top of each other. This way, if you have a relatively homogeneous board (ie. a lot of the same tile type), instead of having to render a new Tile for each Tile in a particular Stack, you can instead have a single Tile but with a height equal to the total number of Tiles at that Point multiplied by the height per tile. To put this in perspective, suppose you have a 10 by 10 by 10 board where every Tile is of type Grass. If the board is full and you render a Tile per Point, you would have to render a total of 10 * 10 * 10 = 1000 tiles. Alternatively, using the optimization described above, since each Point would technically only be rendering one Tile, we would only have to render 10 * 10 = 100 Tiles.
Here is an example board creating using the above board representation in my board editor. It also includes some background assets (water, rocks), as well as environment assets placed on Tiles, such as trees and lights.
Programming TacticsRPG
2019 Mar 02
ciphAR - An Auto-translation app for the Fire Emblem Cipher Card Game
I started collecting cards from the Fire Emblem Cipher card game a few years ago, solely because of the art. I may have gone a little overboard, especially for a game I don't actually play, since I can barely read Japanese.
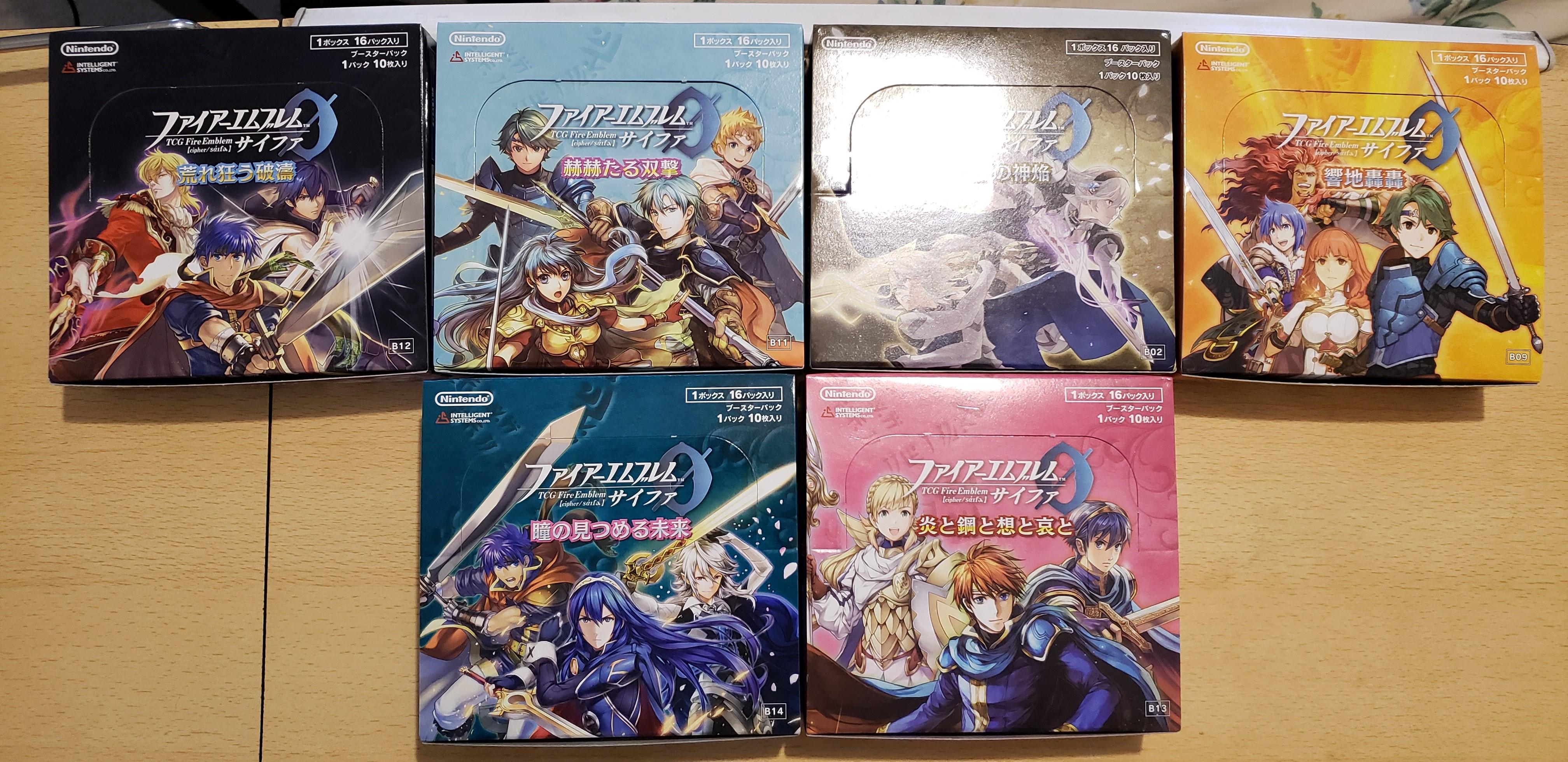
Exhibit 1: A little overboard
To deal with the aforementioned translation issue, I'm developing a small AR application that will scan FE Cipher cards and superimpose the cards' translation on top of them. Here is a sample scanned output:
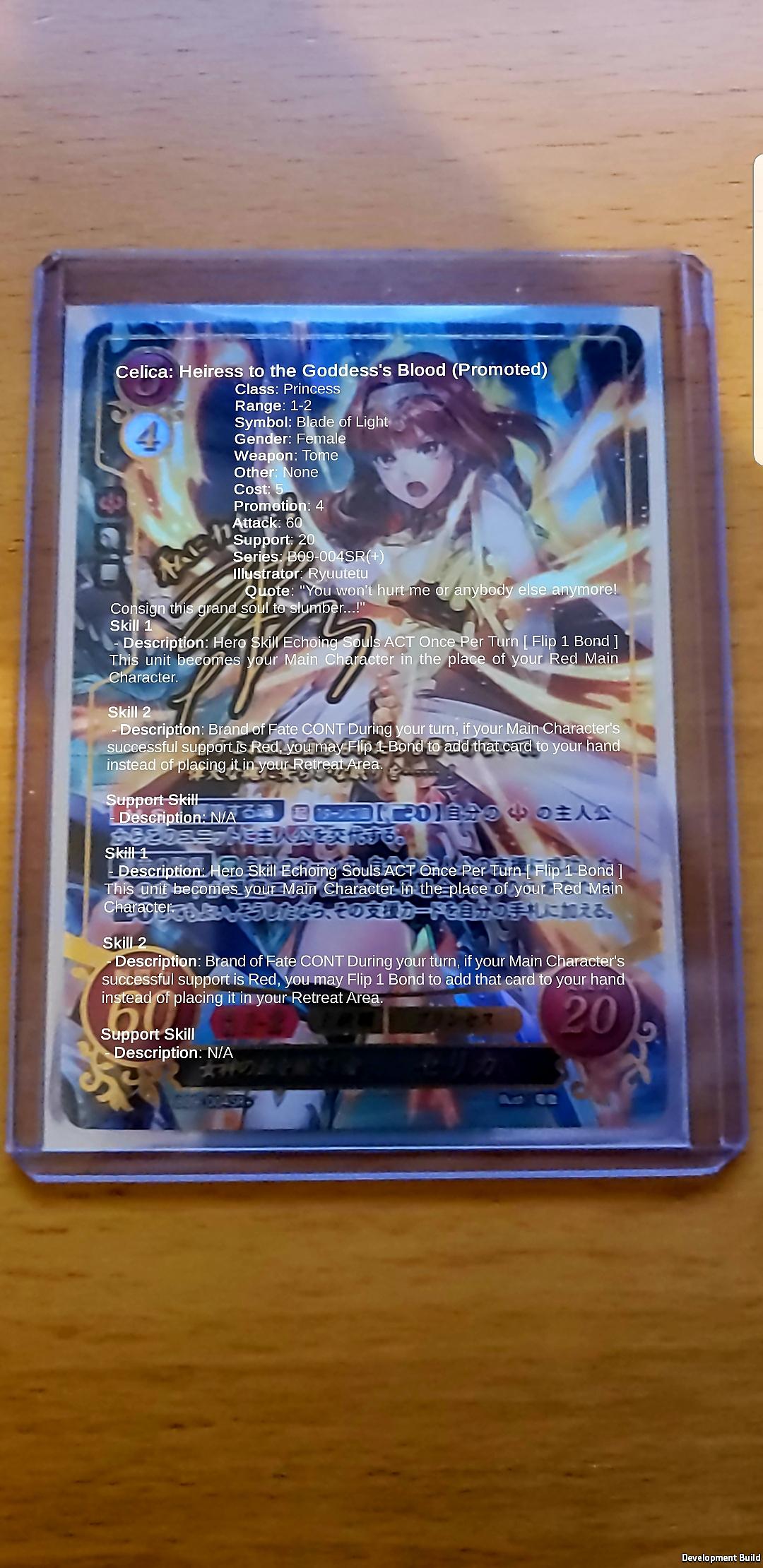
The superimposed text auto-adjusts based camera position, and generally maintains its world-space position unless the user moves the camera far away from the initial scanned position of the card.
I'll probably be releasing the application to android over the next few days, and I'll add features based on feedback.
Programming ciphAR